Classroom Map
An interactive classroom mapping system that helps students and faculty navigate campus buildings and find classrooms efficiently.
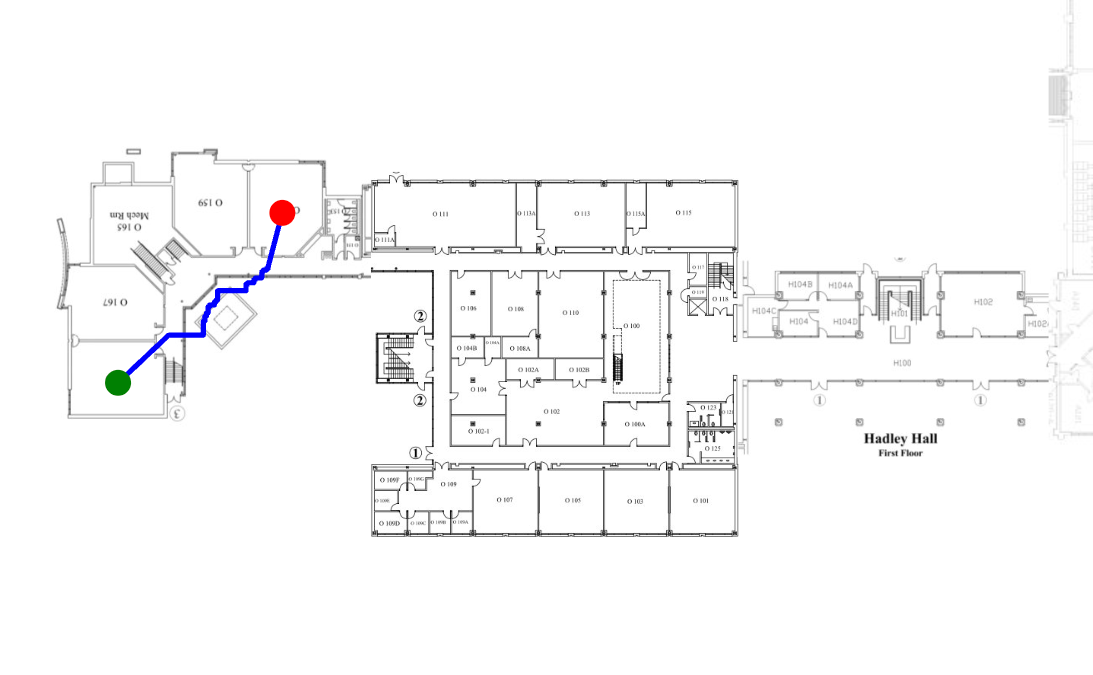
Technologies Used
- • JavaScript
- • Pathfinding
- • Node.js
- • Firebase
- • Express
- • HTML
- • CSS
Key Features
- • Interactive campus map
- • Room search functionality
- • Navigation assistance
- • Personal Schedule Setup
- • Mobile responsive
- • Accessibility features
Project Description
The Classroom Map project is an interactive web application designed to help students and faculty navigate campus buildings and locate classrooms efficiently. It provides a user-friendly interface with real-time updates and accessibility features.
How It Works:
The application uses Pathfinding Algorithm for rendering interactive maps and provides search functionality to find the shortest paths between two points. It features a responsive design that works well on both desktop and mobile devices, with real-time updates for room availability and maintenance status.
Technical Implementation:
- • Interactive map interface
- • Search and filtering system
- • Enabling Firebase for Authentication
- • Personal Schedule Setup
- • Navigation assistance
- • Accessibility features
Technical Challenges
1. Map Performance
Optimizing map rendering and interaction performance for large campus layouts.
2. Pathfinding Algorithm
Implementing the function to find the shortest path between two points based on Pathfinding Algorithm for games.
3. Login and Authentication
Implementing the login and authentication system through Firebase to allow users to save their personal schedules and access them later.
4. Accessibility
Ensuring the application is fully accessible to users with different needs.
Code Snippets
// Setup grid for classrooms
function getDistance(point1, point2) {
return Math.sqrt(Math.pow(point1.x - point2.x, 2) + Math.pow(point1.y - point2.y, 2));
}
function getClosestPathNode(classroom, paths) {
let closestPath;
let shortestDistance = Infinity;
for (let key in paths) {
let path = paths[key];
let distance = getDistance(classroom, path);
if (distance < shortestDistance) {
shortestDistance = distance;
closestPath = path;
}
}
return closestPath;
}
function setupGrid(width, height, paths, walls) {
let grid = new PF.Grid(width, height);
// Check if walls data is defined and is an array before attempting to use it
if (Array.isArray(walls)) {
walls.forEach(wall => {
let points = getLinePoints(wall.start, wall.end);
points.forEach(point => {
grid.setWalkableAt(point.x, point.y, false);
});
});
} else {
console.error('Walls data is not loaded or not an array:', walls);
}
return grid;
}
// Find complete route function
function findPathBetweenNodes(startNode, endNode, grid) {
let finder = new PF.AStarFinder();
return finder.findPath(startNode.x, startNode.y, endNode.x, endNode.y, grid.clone());
}
function findCompleteRoute(classroomA, classroomB, paths,walls) {
let grid = setupGrid(1500,1500,paths,walls);
let startPathNode = getClosestPathNode(classroomA, paths);
let endPathNode = getClosestPathNode(classroomB, paths);
console.log('startPathNode:', startPathNode);
console.log('endPathNode:', endPathNode);
if (!startPathNode || !endPathNode) {
console.error('One of the path nodes is undefined.');
return null;
}
let mainPath = findPathBetweenNodes(startPathNode, endPathNode, grid);
if (!mainPath || mainPath.length === 0) {
console.error('Main path is empty or undefined.');
return null;
}
let completePath = [[classroomA.x, classroomA.y], ...mainPath, [classroomB.x, classroomB.y]];
return completePath;
}